How I over-optimized my site - Part 2: The Core
In this second part of my over-optimization journey, I'll dive into the details of the framework, database, template engine, and other key components that make Bpress tick
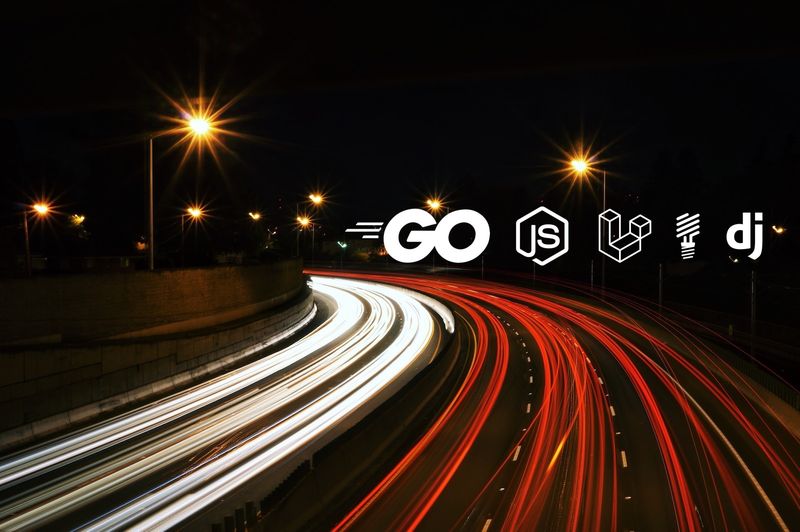
As a programmer obsessed with performance optimization, I decided to build my own Content Management System (CMS) called Bpress. These choices come from my years of using 12 golang web frameworks, 6 template engines in various large and small projects
Framework: Fiber
When it came to choosing a framework for Bpress, I went with Fiber. Fiber is built on top of Fasthttp, which is known for its blazing fast performance. According to the TechEmpower Web Framework Benchmarks (Round 22), Fiber consistently ranks among the top performers in various categories.
Here's a quick comparison of requests per second for JSON Serialization:
- Fiber (prefork): 955,738
- Gin: 406,943
- Spring: 236,259
- Express: 92,604
(Source: https://www.techempower.com/benchmarks/#section=data-r20&hw=ph&test=json)
But it's not just about raw speed. Fiber also offers:
- Express-style routing
- Low memory footprint
- Zero memory allocation (in most cases)
- Rapid development with its intuitive APIAs an efficiency-focused developer, Fiber's performance and ease of use were a perfect match for my needs.
Database: Flexible Approach
I designed Bpress to work seamlessly with MySQL, PostgreSQL, and SQLite. This flexible approach allows me to switch databases as needed.The key is an abstraction layer that handles the database operations, allowing me to swap out the underlying database without significant code changes. It's like having a universal interface for databases - one approach to handle them all.
Jet Template Engine
For templating, I chose Jet. Jet is one of the fastest template engines available for Go. It compiles templates to native Go code, resulting in very fast execution. In benchmarks, Jet consistently outperforms other popular template engines like html/template and text/template.Here's a quick comparison of template execution times:
- Jet: 0.339 ms
- html/template: 1.578 ms
- text/template: 1.441 ms
(Source: https://github.com/CloudyKit/jet#benchmarks)
Plus, Jet's syntax is clean and intuitive, making it enjoyable to work with. It's like the Go of template engines - fast, simple, and efficient.
Bleve: Full-Text and Vector Indexing
For full-text indexing and vector indexing to support LLM-related tasks, I turned to Bleve. It's like having a search engine and a vector database combined into one powerful tool. Bleve offers:
- Full-text search capabilities
- Vector indexing for semantic search
- Flexible indexing options
- Support for multiple languagesWith Bleve, I can handle everything from simple keyword searches to complex semantic queries efficiently.
Static Files and HTML Optimization
Static files and HTML documents in Bpress go through an optimization process before being served:
- Minification: Removing unnecessary whitespace and comments.
- Caching: Storing frequently accessed files in memory for quick retrieval.The result is smaller, faster-loading files that improve overall site performance.
JS and CSS: On-Demand Serving
In Bpress, JavaScript and CSS are served on-demand based on the page context. This approach:
- Reduces unnecessary code bloat
- Improves page load times
- Minimizes bandwidth usage, ensures that users only receive the code they actually need for the current page.
Image Optimization
For image serving, I built an image proxy server (without the need of URL signatures). The system can:
- Detects browser compatibility for next-gen image formats
- Serves WebP or AVIF images when supported
- Falls back to traditional formats for older browsers
Images are resized and optimized on-the-fly, ensuring that users get the optimal balance of quality and performance.
Building Bpress has been an exercise in optimization. From the high-performance Fiber framework to the efficient image serving system, every component has been chosen and configured for maximum performance.
While it might be over-engineered for some use cases, the process has been both educational and rewarding. The end result is a CMS that's finely tuned for speed and efficiency.
Stay tuned for the next part of this series, where I'll explore the frontend optimizations that further enhance Bpress's performance. Until then, keep optimizing!