The Actor Model in Game Development
When designing game servers to leverage multi-core processors, I often choose between two main architectures: single-threaded multi-process and single-process multi-threaded. Each approach has its own set of advantages and challenges
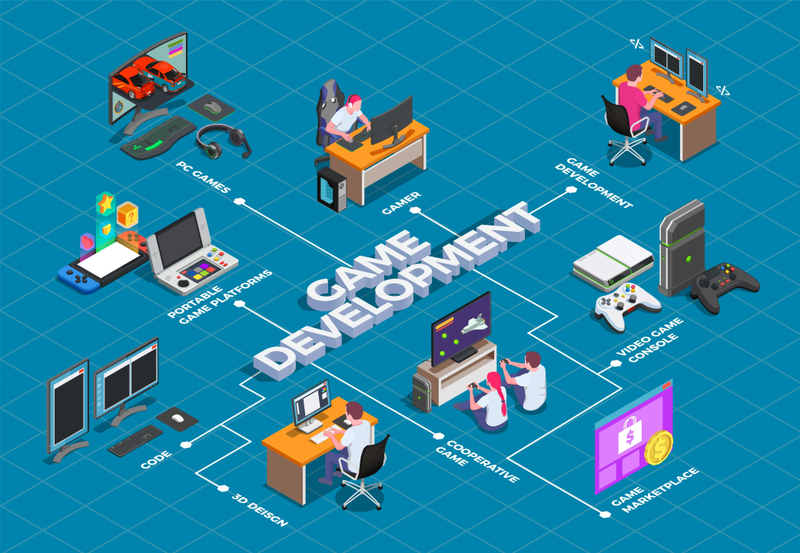
While the Actor Model provides a conceptual framework for concurrent and distributed systems, its implementation in game development can vary based on the underlying architectural approach. Two primary architectures are commonly used in game development: single-threaded multi-process and single-process multi-threaded. Each approach has its own set of advantages and trade-offs, particularly in the context of game engines.
Single-Threaded Multi-Process Architecture
This approach runs multiple single-threaded processes, each potentially acting as an independent actor or handling a specific game subsystem.
Advantages:
- Better fault isolation: If one process crashes, it doesn't affect others, enhancing overall system stability.
- Easier to scale across multiple physical machines: There's little difference between running on one machine or many, which is beneficial for distributed game servers.
- Utilizes existing profiling tools: Standard system tools like
top
can be used to monitor CPU and memory usage of individual processes, simplifying debugging and optimization. - Avoids race conditions and deadlocks between processes: Each process has its own memory space, eliminating shared state issues.
Disadvantages:
- Higher resource usage due to separate memory spaces for each process.
- Inter-process communication requires serialization and deserialization of messages, which can impact performance in high-frequency communication scenarios common in games.
- Slight latency when sending messages between processes, which can be critical in fast-paced games.
Single-Process Multi-Threaded Architecture
This approach runs multiple threads within a single process, with each thread potentially managing multiple actors or game subsystems.
Advantages:
- Lower resource usage since threads share memory within the same process.
- Faster inter-actor communication within the same process, which can be crucial for game logic that requires frequent interactions.
- Can fully utilize multi-core processors on a single machine more efficiently, which is important for modern game engines.
Disadvantages:
- Bugs in one thread can potentially crash the entire game process.
- Requires careful synchronization to avoid race conditions and deadlocks, which can be challenging in complex game systems.
- More complex to debug and reason about due to shared state, which can slow down development and make it harder to maintain game code.
The Actor Model in Game Development
In the context of game development, the Actor Model can be applied in various ways to manage game entities and systems. A game actor is typically an object that represents a single entity in the game world, such as a character, item, or environmental element.The Actor Model in games can help manage the complexity of game systems by encapsulating state and behavior within individual actors. This approach can be particularly useful for:
- Managing NPCs and AI behavior
- Handling player interactions and state
- Coordinating game world events and physics simulations
- Managing networking and multiplayer synchronizationHowever, it's important to note that many games do not fully implement the Actor Model in its purest form. Instead, they often adopt hybrid approaches that combine aspects of the Actor Model with other architectural patterns like Entity-Component Systems (ECS).
Case Study: Halo 4 and the Actor Model
A notable example of the Actor Model's application in game development is the case of Halo 4. When developing this game, the team at Microsoft Game Studios faced significant challenges in meeting the requirements for online play, scalability, and performance.To address these challenges, they implemented a solution based on the Actor Model using the Orleans framework. This approach allowed them to:
- Handle massive player loads (1 million unique players on launch day, 4 million within a week)
- Ensure high availability for online play
- Achieve low latency and high concurrency
- Create stateful services with the performance benefits of a cache but without concurrency issuesThe use of the Actor Model, implemented through Orleans, enabled the Halo 4 team to sustainably run above 90% CPU utilization on their servers with nearly linear scalability. This was particularly valuable for adapting to dramatic load changes, such as during the game's launch.
Considerations for Game Developers
When deciding between single-threaded multi-process and single-process multi-threaded architectures, or considering the implementation of the Actor Model, game developers should consider:
- The scale and complexity of the game world
- The required level of concurrency and parallelism
- The target platforms and their hardware capabilities
- The team's expertise in concurrent programming and debugging
- The specific performance requirements of different game subsystems
It's worth noting that many modern game engines use hybrid approaches, combining elements of both architectures and selectively applying Actor Model concepts where they provide the most benefit. For example, they might use multi-threading for certain subsystems like physics or AI, while keeping the main game loop single-threaded for simplicity and determinism.In conclusion, while the Actor Model and multi-threaded architectures offer powerful tools for managing complexity and improving performance in game development, their implementation must be carefully considered in the context of each game's specific requirements and constraints.