Game exploiting using function detours
Function detouring is a technique used to intercept and modify the behavior of existing functions in a running process. This can be particularly useful for modifying game behavior, adding new features,...
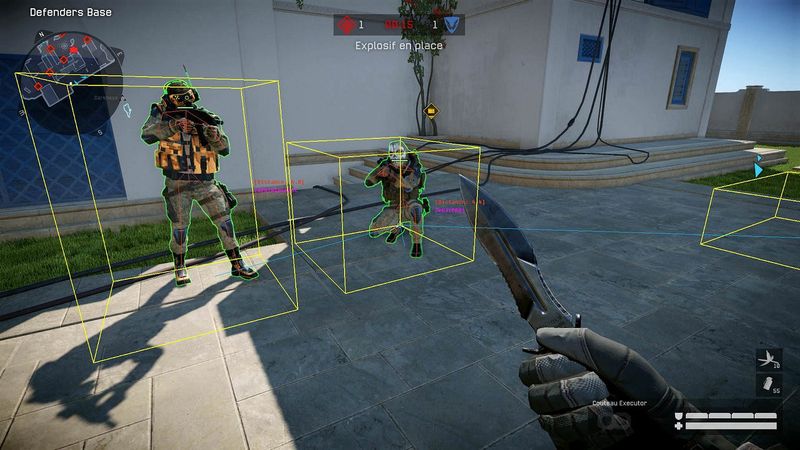
Function detouring is a technique used to intercept and modify the behavior of existing functions in a running process. This can be particularly useful for modifying game behavior, adding new features, or fixing bugs in games where you don't have access to the source code.
Define the Original Function Signature
Identify the function you want to detour and define its signature. For this example, let's assume we're detouring a function that calculates player health:
[UnmanagedFunctionPointer(CallingConvention.Cdecl)]
delegate int CalculateHealthDelegate(int baseHealth, int bonusHealth);
Implement the Detour Function
Create a method that will replace the original function:
static int CalculateHealthHook(int baseHealth, int bonusHealth)
{
// Your custom logic here
Console.WriteLine($"Original health calculation: {baseHealth + bonusHealth}");
return (baseHealth + bonusHealth) * 2; // Double the health for this example
}
Create the Main Hook Class
public class GameHook : IEntryPoint
{
private static LocalHook _calculateHealthHook;
public GameHook(RemoteHooking.IContext context)
{
// Constructor
}
public void Run(RemoteHooking.IContext context)
{
try
{
// Get the address of the original function
IntPtr calculateHealthAddress = GetFunctionAddress("GameAssembly.dll", "CalculateHealth");
// Create and install the hook
_calculateHealthHook = LocalHook.Create(
calculateHealthAddress,
new CalculateHealthDelegate(CalculateHealthHook),
null);
// Activate the hook
_calculateHealthHook.ThreadACL.SetExclusiveACL(new int[1]);
Console.WriteLine("Hook installed successfully");
// Keep the DLL loaded
while (true)
{
System.Threading.Thread.Sleep(100);
}
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
// Helper method to get function address
[DllImport("kernel32.dll", CharSet = CharSet.Ansi, SetLastError = true)]
static extern IntPtr GetProcAddress(IntPtr hModule, string procName);
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
public static extern IntPtr GetModuleHandle(string lpModuleName);
static IntPtr GetFunctionAddress(string moduleName, string functionName)
{
IntPtr moduleHandle = GetModuleHandle(moduleName);
return GetProcAddress(moduleHandle, functionName);
}
}
Then build your project to create the DLL.
Inject the DLL into the Game Process
To inject the DLL, you'll need a separate injector application. Here's a simple example using EasyHook:
using System;
using EasyHook;
class Injector
{
static void Main(string[] args)
{
try
{
int targetPID = 1234; // Replace with actual game process ID
string dllPath = @"C:\Path\To\Your\GameHookDLL.dll";
RemoteHooking.Inject(
targetPID,
InjectionOptions.Default,
dllPath,
dllPath,
// any parameters you want to pass to your DLL
);
Console.WriteLine("DLL injected successfully");
}
catch (Exception ex)
{
Console.WriteLine($"Injection failed: {ex.Message}");
}
}
}
This tutorial provides a basic framework for detouring functions in a game process using C# and DLL injection. Remember that modifying game processes may violate terms of service for many games, especially in multiplayer environments. Always use this technique responsibly and ethically.Also, note that anti-cheat systems in modern games may detect and prevent such modifications. This method is more suitable for educational purposes or for modifying single-player games where you have permission to do so.