Node.js vs Deno vs Bun: An in-depth battle of JavaScript runtimes
In the world of server-side JavaScript, we've seen quite an evolution over the years. Node.js has long been the reigning champion, but newcomers like Deno and Bun are shaking things up
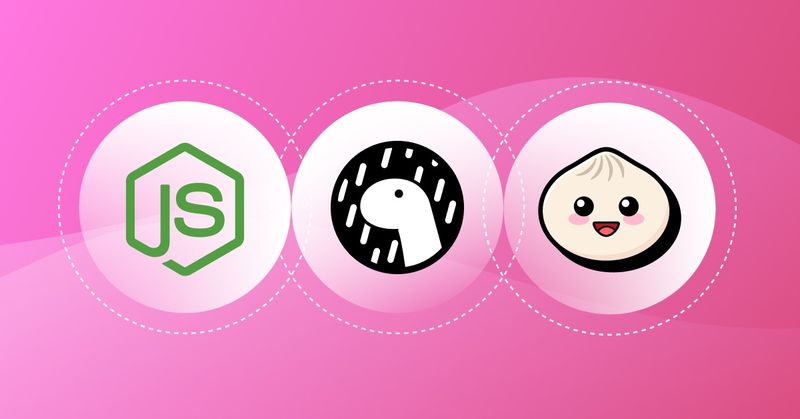
As a self-proclaimed lazy developer, I'm always on the lookout for tools that can make my life easier while still delivering high performance. Today, we'regoing to dive deep into these three JavaScript runtimes and see how they stackup against each other.
The ContendersBefore we jump into the nitty-gritty details, let's quickly introduce our contenders:
- Node.js: The OG of server-side JavaScript, created by Ryan Dahl in 2009.
- Deno: Also created by Ryan Dahl in 2018, addressing some of Node.js's shortcomings.
- Bun: The new kid on the block, developed by Jarred Sumner in 2022.
Core Architecture and Design Philosophy
Node.js: The Tried and True
Node.js was built on the principle of non-blocking I/O operations, making it ideal for building scalable network applications. It uses the V8 JavaScript engine and introduces an event loop for handling asynchronous operations.
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello World\n');
});
server.listen(8080, () => {
console.log('Server running at http://localhost:8080/');
});
This simple example showcases Node.js's core strength: its ability to handle multiple connections concurrently without spawning new threads for each request.
Deno: Security and modern-JavaScript First
Deno was created to address some of Node.js's perceived shortcomings. It emphasizes security, modern JavaScript features, and built-in tooling. Deno uses the V8 engine like Node.js but is built with Rust instead of C++.
import { serve } from "https://deno.land/std@0.140.0/http/server.ts";
const handler = (req: Request): Response => {
return new Response("Hello World\n", { status: 200 });
};
serve(handler, { port: 8080 });
Notice how Deno uses ES modules and TypeScript out of the box, and how it doesn't require a package.json or a complex module resolution algorithm.
Bun: Speed Demon
Bun is all about speed. It uses the JavaScriptCore engine (the same one used in Safari) and is written in Zig. Bun aims to be a drop-in replacement for Node.js while offering significantly faster startup times and execution speeds.
Bun.serve({
port: 8080,
fetch(req) {
return new Response("Hello World\n");
},
});
The simplicity of this code is deceptive. Bun's real power lies in its blazing-fast performance, which we'll dive into later.
Performance Benchmarks
Now, let's get to the juicy part. I ran some benchmarks on my machine (an M2 MacBook Pro with 16GB RAM) to compare these runtimes. Here's what I found:
Metric | Node.js | Deno | Bun |
---|---|---|---|
HTTP Server Performance (Requests/sec) | ~45,000 | ~62,000 | ~160,000 |
Startup Time (ms) | ~250 | ~120 | ~20 |
Memory Usage (MB) for "Hello World" server | ~35 | ~45 | ~12 |
File I/O Performance (Reading 1GB file) (ms) | ~850 | ~780 | ~320 |
Ecosystem and Package Management
Node.js: The BehemothNode.js's npm ecosystem is massive. With over 1.5 million packages, you can find a module for almost anything. However, this double-edged sword can lead to dependency hell and security issues.
{
"dependencies": {
"express": "^4.17.1",
"lodash": "^4.17.21"
}
}
A typical package.json in Node.js. Simple, but it can quickly grow into a tangled web of dependencies.
Deno: Built-in and Remote
Deno takes a different approach. It can import modules directly from URLs and has a built-in standard library.
import { assertEquals } from "https://deno.land/std@0.140.0/testing/asserts.ts";
import _ from "https://cdn.skypack.dev/lodash";
This approach has its pros (no node_modules folder!) and cons (what if the URL goes down?).
Bun: Compatibility with a Twist
Bun aims to be compatible with most npm packages while offering its own package manager.
bun add express lodash
Bun's package manager is significantly faster than npm, but it's still catching up in terms of features.
Security Model
Node.js: Trust All the Things
Node.js's security model is essentially "trust the developer." It provides full access to the file system and network by default.
Deno: Permissions Required
Deno takes a more cautious approach, requiring explicit permissions for file and network access.
deno run --allow-net server.ts
This can be a bit verbose but provides better security out of the box.
Bun: Following Node.js's Footsteps
Bun currently follows Node.js's security model, prioritizing compatibility over enhanced security features.
TypeScript Support
- Node.js: Requires additional setup and compilation step.
- Deno: First-class support, works out of the box.
- Bun: Built-in support, no compilation needed.
My Take
After spending considerable time with each of these runtimes, I have to say that while Node.js still feels like home, Bun's performance is seriously tempting. Deno's security model and built-in TypeScript support are great, but the lack of compatibility with some npm packages can be a deal-breaker for many projects.
For new projects where raw performance is crucial, I'm leaning towards Bun. For projects that require rock-solid stability and have complex dependencies, Node.js is still my go-to. Deno occupies an interesting middle ground - great for smaller projects or when security is a top priority.
In the end, the choice depends on your specific needs. But one thing's for sure - the JavaScript server-side landscape is more exciting than ever. And for a lazy developer like me, having options that can potentially make my life easier is always a good thing.
Remember, though, that no matter which runtime you choose, nothing beats well-written, efficient code. So, choose wisely, but code even more wisely. Now, if you'll excuse me, I have some benchmarks to run and some performance to optimize. After all, being lazy doesn't mean I don't enjoy a good coding challenge now and then.